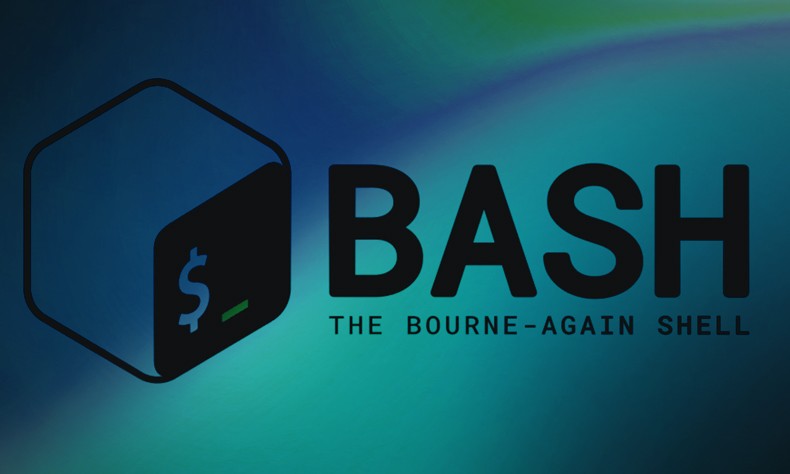
What is a Regular Expression (Regex)?
The term Regex or in full – regular expression, refers to a sequence of characters that form a particular search pattern. These expressions allow you to match, search, and manipulate text based on specific patterns. Regular expression is supported in many programming languages and tools, including Bash.
Why Use Regex in Bash Scripts?
Using regex in your bash script has a number of benefits Such benefits include:
1. Text Search and Manipulation: Regex makes it easy to search for specific patterns in text data, enabling advanced text processing and manipulation.
2. Input Validation: You can use regex to validate user input or data formats, ensuring that your scripts work with correctly formatted data.
3. File Operations: Regex can help you filter, rename, or process files based on their names or contents.
4. String Manipulation: Bash’s inbuilt string manipulation tools can be combined with regex for powerful text processing capabilities.
Bash Regex – The Basic Syntax
Regex, which stands for regular expressions, can be used in conditionals and with commands like grep, sed, and awk in Bash scripting. The core linguistic structure of regex in Bash is similar to any other programming language, however it is important to remember that certain characters might require a punctuation line (\) before them.
YouTube Link: Regular Expressions (RegEx) Tutorial #2 – Simple RegEx Patterns
Here are Some of the Commonly Used Regex Elements in Bash:
– `.` matches any single character except a newline
– `\d` matches any digit character
– `\w` matches any word character (alphanumeric and underscore)
– `^` matches the start of a line
– `$` matches the end of a line
Here Are Some of The Commonly Used Regex Patterns
Some of the widely used regex patterns in Bash scripts include;
– `^` matches lines starting with a hash symbol (comments in many programming languages)
– `^\s$` matches blank lines
– `\b\w+\b` matches whole words
– `\d{4}-\d{2}-\d{2}` matches dates in the format YYYY-MM-DD
– `\w+@\w+\.\w+` matches simple email addresses
How to Use Regex with Bash Commands
Regex in Bash is often used in combination with commands like `grep`, `sed`, and others. Here are some examples:
How to Use Regex for Character Classes
There are several character classes that allow you to match a specific set of characters. Here are some common character classes in Bash regex:
– `[abc]` matches any single character within the brackets
– `[^abc]` matches any single character not within the brackets
– `[a-z]` matches any lowercase letter
– `[A-Z]` matches any uppercase letter
– `[0-9]` matches any digit
Regex Quantifiers
Quantifiers specify how many times a pattern should be matched. Here are some common quantifiers in Bash regex:
– `?` matches the preceding element zero or one time
– “ matches the preceding element zero or more times
– `+` matches the preceding element one or more times
– `{n}` matches the preceding element exactly `n` times
– `{n,}` matches the preceding element at least `n` times
– `{n,m}` matches the preceding element between `n` and `m` times
Regex Grouping and Capturing
Characters such as parentheses `()` can be used to group patterns in regex. This allows you to apply quantifiers or perform capturing for later use. i.e.helps you to repeat or remember specific parts of the text you’re looking for.
Regex Lookarounds
Lookarounds allow you to match patterns based on the presence or absence of other patterns around them, without including those patterns in the match. Bash supports positive and negative lookaheads (`(?=pattern)` and `(?!pattern)`). Regex lookarounds are like secret agents checking the surroundings before making a move. In Bash, you can use them to see if certain patterns are nearby without actually grabbing them. It’s like peeking ahead and saying “okay, proceed” or “abort mission” without getting tangled up in the details.
Regex Substitution and Replacement
Regular expressions in Bash aren’t just limited to matching and searching text, In simple terms, besides finding and searching for specific text, regular expressions in Bash can also help you swap and change text patterns. This is really useful when you need to make big changes to lots of text or tidy up messy data.
One popular tool for this in Bash is called ‘sed’ (Stream EDitor). It’s like a magic wand for replacing text using regular expressions. For example, you can use sed to swap out every instance of a certain word or phrase with a new one.
With ‘sed,’ you can do even more cool stuff by capturing parts of the text you find and using them in the new text you’re putting in. It’s like cutting out a piece of a picture and pasting it somewhere else. For example:
In Bash, there’s another handy tool called the =~ operator that lets you use regular expressions to compare patterns in if statements and similar commands. It’s like having a special filter that helps you decide what to do based on how something looks or fits together.
Advanced Regex Techniques
Once you’ve mastered the basics of regex, there are some cool advanced techniques you can learn in Bash. These techniques give you even more power to manipulate and work with text in creative ways.
Negative Lookbehind
In addition to negative lookahead ((?!pattern)), Negative Lookbehind is another tool in Bash regex that’s pretty handy. It’s like checking behind a word to see if something specific isn’t there before deciding if it’s a match. This helps you exclude certain matches based on what comes before them in the text.
Recursive Patterns
Bash supports recursive patterns, you can find patterns within patterns, kind of like finding smaller puzzles inside a bigger puzzle. This is really handy when dealing with data that’s organized in layers, like when you’re working with complex files or documents.
Regex Backreferences
Regex backreferences let you refer back to and reuse bits of text you’ve already found in your pattern. It’s like using a shortcut to repeat something you’ve already said. This can be handy for making sure your pattern matches all the right parts and stays consistent throughout.
Regex Comments
In Bash, you can add comments within your regex patterns using the syntax (?#comment). It’s like leaving little reminders or explanations right inside your pattern, which can be really useful for keeping track of what each part of the pattern is doing, especially when it gets complicated.
Best Practices for Regex in Bash
When using regex in Bash scripts, it is important to consider the following practices that are considered industry’s standard.
1. Test Your Regex: before using them in the scripts, the use of online regex testers or tools like `grep -E` to validate your regular expressions has to be done.
2. Use Comments and Documentation: Write clear explanations for your regex patterns, especially if they’re complex in nature. This helps you and others understand what each part does.
3. Escape Special Characters: Minimize the use of special characters in regex patterns to avoid unintended matches or errors. i.e. Don’t forget to put a backslash (\) before special characters in your regex. This prevents them from causing unexpected matches or errors.
4. Consider Performance: While regex is great, it can slow things down if you’re working with big data or complicated patterns. Try to make your regex efficient, and if it’s causing problems, think about other ways to do things.
5. Use Modular Design: Break your regex into smaller pieces or bits that you can reuse. This makes your code easier to understand and maintain.
6. Checking User Input: If you’re using regex to check what people type in, think about all the weird things they might try and give them helpful error messages if they get it wrong.
In short, learning how to use regex well in Bash gives you a powerful way to work with text, manipulate data, and check inputs in your scripts.
Internal Links:
External References: