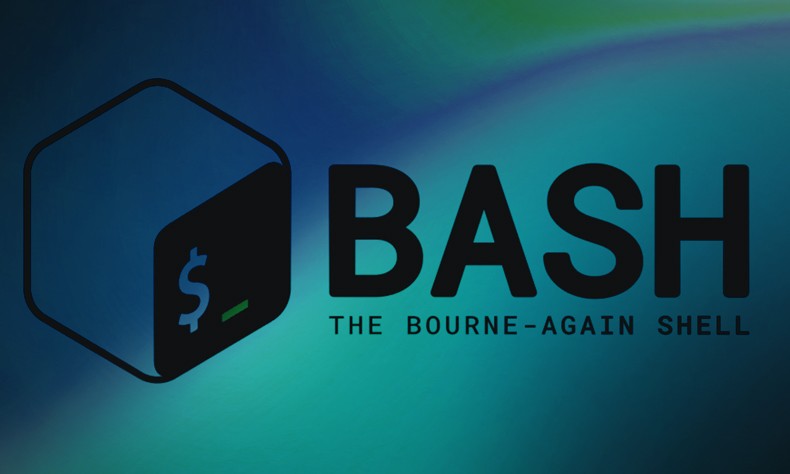
When I first delved into bash scripting, arguments seemed like a mystery, holding the key to unlocking the language of code.
During a late-night coding session, I realized their power while crafting a script for file management. By utilizing arguments to dynamically pass directory paths, my script became flexible and responsive, transforming a rigid tool into a dynamic asset.
What is a Bash Script?
A Bash script can be defined as a plain text file containing a series of commands that can be executed by the Bash shell, which is the default shell on most Linux distributions and macOS. Bash (Bourne Again SHell) is a command-line interpreter that reads and interprets the commands written in the script file.
Bash scripts have various functionalities and uses. These mainly include automating various tasks and system administration tasks on Unix-like operating ‘systemsy’. Bash scripts also allow you to combine multiple commands, control the flow of execution using conditional statements and loops, and perform complex operations with minimal user interaction.
Youtube Link: Bash Scripting on Linux
What are Some of the Use Cases of Bash Script?
Here are some key features and use cases of Bash scripts:
- Automation: Bash scripts can automate repetitive tasks, such as file management, system backups, software installations, and data processing.
- System Administration: System administrators often use Bash scripts for tasks like user management, system monitoring, log analysis, and server configuration.
- Script Portability: Bash scripts are portable across different Unix-like systems, making them useful for managing and administering multiple servers or environments.
- Complex Operations: With Bash scripting, you can perform complex operations by combining various commands, utilities, and external programs.
- Text Processing: Bash scripts are particularly useful for text processing tasks, such as parsing log files, manipulating data files, and generating reports.
- Command-line Interfaces (CLIs): Bash scripts can be used to create interactive command-line interfaces for applications or tools, providing a user-friendly way to execute complex operations.
- Scheduling Tasks: Bash scripts can be scheduled to run at specific times or intervals using cron jobs, making them useful for automating regular maintenance tasks.
- Environment Setup: Bash scripts are often used to set up development environments, install dependencies, and configure software on various platforms.
Bash scripts vary from short commands i.e simple one-liners to detailed programs with progressed features like functions and arrays. They can connect with other languages like Python or Ruby, enabling more advanced scripting options.”
What Are Bash Script Arguments?
Just like any other programming or scripting languages Bash script arguments are similar. They refer to the values passed to a bash script when it is executed. Arguments in your Bash script will allow you to provide dynamic input or configuration to your scripts, making them more flexible and reusable.
Why Should You Use Arguments in Bash Scripts?
Reasons as to why you might want to use arguments in your bash scripts include:
- Increased Flexibility: By incorporating arguments in your script, allows for the ability to execute various tasks or handle diverse data sets, depending on the input received.
- Code Reusability: Rather than embedding values directly into your script, passing them as arguments facilitates the reuse of the same script for multiple objectives.
- User Interaction: It allows for users to actively relate with the script and give their preferred input or suggestions
How to Pass Arguments to a Bash Script
To pass arguments to a bash script, you simply need to provide them after the script’s filename when executing it. Here’s the basic syntax:
You can pass as many arguments as needed, separated by spaces.
Youtube Link: Bash Script Arguments
Accessing Arguments Inside the Script
Within your bash script, you can access the provided arguments using special variables:
– `$0` represents the script’s filename.
– `$1` refers to the first argument, `
– ‘$2` to the second argument, and so on.
– `$` holds the total number of arguments passed to the script.
– `$@` represents all the arguments as a single entity.
Here’s an example that demonstrates how to access and use these variables:
Dealing with Varied Numbers of Inputs
It’s wise to prepare for situations where the number of inputs given doesn’t match what your script needs. You can use special commands and symbols like $ to check how many inputs there are and adjust your script accordingly.
If the script doesn’t get exactly two inputs i.e. arguments, a message on how to use it and stop running with a signal that something went wrong will be shown.
Arguments vs Options
In simple terms, while arguments are values given in a certain order to the script, options are marked with a hyphen (-) and are usually used to change how the script works or add extra settings.
Here’s an example of using both arguments and options:
In the above code snippet, the script has two choices you can pick: -h to see help and -o to say where you want the output to go. It also needs two things in a certain order called arg1 and arg2.
Figuring Out Tough Arguments
When you’re dealing with simple variables, just using $1, $2, and so on to grab the arguments is fine. But for trickier scripts, you might need smarter ways to understand and manage the inputs. This could include things like:
- Arguments that have spaces or funny symbols
- Choices that you don’t have to pick, but have a default
- Different types of choices (like numbers or words)
- Choices that rely on each other, or ones you can’t pick together
For these situations, you can lean on tools like “getopts” or special libraries that help sort out the tricky arguments.
Using ‘getopts‘
“getopts” is like a built-in helper in Bash. It helps you figure out those short choices (like just one letter with a hyphen in front) and what goes with them. Here’s how you can use it:
By Using External Libraries
Sometimes arguments can be too complex to be parsed by bash script alone. In this case you can always consider using external libraries or frameworks. These libraries are specifically designed for parsing and validating command-line arguments in Bash scripts. Some popular options include:
- argumentparser: A powerful argument parsing library for Bash scripts, providing support for long options, different data types, and advanced validation rules.
- argbash: A Bash argument parsing library that generates the argument parsing code based on a declarative syntax, making it easier to define and maintain complex argument structures.
- bash-argparse: Another argument parsing library for Bash scripts, offering support for long options, argument groups, and automatic help generation.
One reason to consider external libraries is because they typically provide more advanced features, such as option grouping, automatic help generation, and better argument validation capabilities. This makes them suitable for complex scripting scenarios.
Best Practices for Using Arguments
Here are some tips to keep in mind when working with arguments in your bash scripts:
- Validate Input: Authenticate and substantiate the arguments then make sure the inputs you get make sense for what your script needs, and handle any weird or wrong inputs gracefully.
- Provide Usage Information: Give clear instructions on how to use your script and what inputs it’s looking for.
- Use Descriptive Argument Names: use specific and descriptive names to explain what each argument represents. If your script needs certain types of inputs (like file names or web addresses), give them names that make it obvious what they’re for.
- Consider Default Values: Help users execute your script more easily by providing default values for parameters that have sensible defaults so they don’t have to provide every argument every time. If some inputs usually stay the same, you can set them as defaults. This way, users don’t have to type them in every time.
- Document Your Script: Put comments in your script that explain what each input does. It’ll help anyone reading your code, including yourself in the future.
By adhering to these principles and recommended methods, you can develop reliable and user-friendly bash scripts that make the most of arguments, allowing for greater adaptability and the ability to reuse code effectively.
Related Internal Links:
External References: